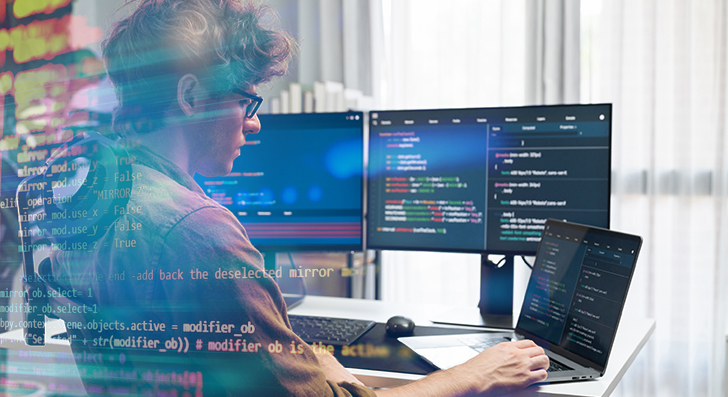
Scalability suggests your software can take care of progress—much more users, extra knowledge, and a lot more site visitors—with out breaking. To be a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and useful guideline that may help you get started by Gustavo Woltmann.
Layout for Scalability from the beginning
Scalability isn't really some thing you bolt on later on—it should be aspect of your plan from the beginning. Several purposes fall short every time they expand fast due to the fact the first design and style can’t tackle the additional load. As being a developer, you'll want to Believe early regarding how your system will behave under pressure.
Start out by creating your architecture to get adaptable. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular style and design or microservices. These styles break your app into scaled-down, impartial sections. Every module or provider can scale By itself with out impacting The full method.
Also, think of your databases from day one particular. Will it have to have to handle 1,000,000 people or merely 100? Choose the appropriate sort—relational or NoSQL—dependant on how your info will grow. System for sharding, indexing, and backups early, Even when you don’t require them nevertheless.
A different vital point is to stop hardcoding assumptions. Don’t write code that only is effective below latest conditions. Contemplate what would happen In case your consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that help scaling, like message queues or occasion-driven techniques. These support your application tackle additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you're reducing upcoming problems. A very well-prepared program is easier to take care of, adapt, and improve. It’s superior to organize early than to rebuild afterwards.
Use the appropriate Database
Selecting the right databases can be a crucial part of setting up scalable apps. Not all databases are designed precisely the same, and using the Erroneous one can gradual you down or maybe result in failures as your app grows.
Start out by comprehension your information. Is it really structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are solid with relationships, transactions, and regularity. Additionally they assistance scaling approaches like study replicas, indexing, and partitioning to take care of a lot more traffic and facts.
In case your facts is more versatile—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured info and will scale horizontally much more easily.
Also, take into account your browse and compose designs. Are you carrying out numerous reads with fewer writes? Use caching and browse replicas. Will you be handling a significant write load? Explore databases that could tackle higher compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent info streams).
It’s also sensible to Assume in advance. You might not need Sophisticated scaling characteristics now, but picking a databases that supports them suggests you received’t need to change later on.
Use indexing to hurry up queries. Prevent unwanted joins. Normalize or denormalize your details depending on your access patterns. And usually keep track of database overall performance while you improve.
To put it briefly, the ideal databases relies on your application’s framework, velocity desires, And just how you be expecting it to increase. Take time to select sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny hold off adds up. Poorly written code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop economical logic from the beginning.
Commence by creating clean up, simple code. Stay clear of repeating logic and take away everything unwanted. Don’t select the most complicated Alternative if an easy 1 works. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to locate bottlenecks—sites the place your code requires too very long to run or works by using a lot of memory.
Up coming, look at your database queries. These often sluggish things down a lot more than the code alone. Be sure each query only asks for the info you actually will need. Keep away from SELECT *, which fetches almost everything, and instead decide on specific fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across massive tables.
For those who recognize exactly the same knowledge remaining requested over and over, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat high priced functions.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra effective.
Remember to take a look at with substantial datasets. Code and queries that work good with 100 documents could possibly crash once they have to deal with 1 million.
In brief, scalable apps are rapidly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques enable your software stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more visitors. If every little thing goes via a single server, it's going to rapidly turn into a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server undertaking many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to build.
Caching is about storing knowledge temporarily so it might be reused swiftly. When customers ask for the identical information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You are able to provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the consumer.
Caching reduces database load, increases speed, and can make your application a lot more economical.
Use caching for things that don’t transform often. And constantly make sure your cache is up to date when details does alter.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app manage additional users, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to get components or guess long run ability. When website traffic improves, you could increase extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and every little thing it must operate—code, libraries, settings—into a person device. This makes it easy to maneuver your app in between environments, from a laptop computer to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, applications like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You may update or scale elements independently, which is great for performance and dependability.
In short, working with cloud and container tools suggests you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, commence working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not correcting.
Watch Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by monitoring standard metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just monitor your servers—keep track of your app as well. Keep watch over how long it will take for consumers to load webpages, how often mistakes take place, and in which they arise. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the response time goes previously mentioned a limit or a service goes down, you should get notified straight away. This allows you deal with troubles rapidly, usually prior to users even see.
Checking is additionally helpful if you make adjustments. In the event you deploy a completely here new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without the need of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
To put it briefly, checking aids you keep the app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it really works properly, even stressed.
Last Views
Scalability isn’t just for major businesses. Even smaller apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper instruments, you are able to Make applications that improve smoothly without having breaking stressed. Start modest, Imagine large, and Create good.