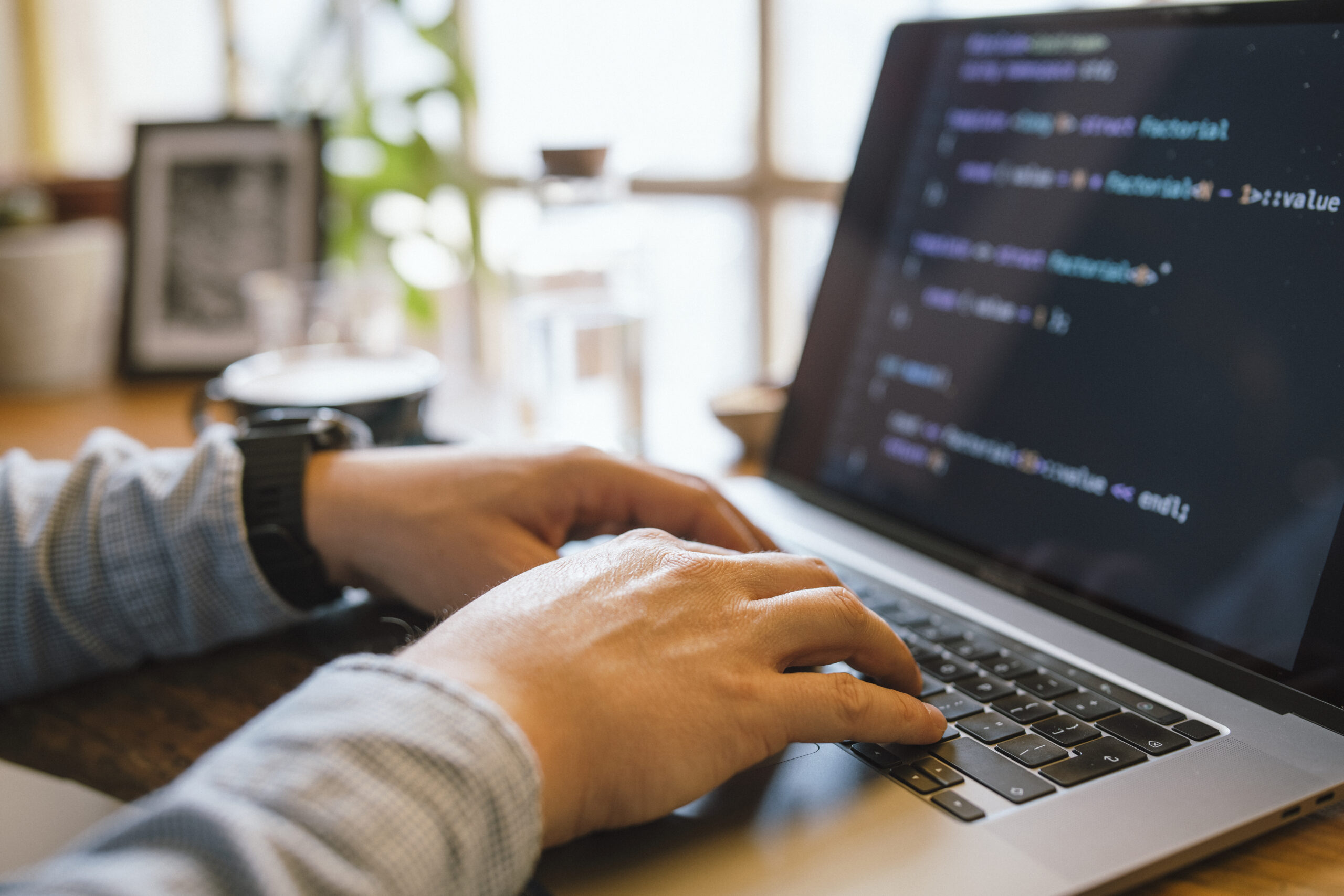
Debugging is Just about the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's not just about fixing broken code; it’s about knowing how and why items go Mistaken, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a rookie or maybe a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and significantly enhance your productiveness. Listed below are numerous techniques to assist developers amount up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging expertise is by mastering the resources they use every day. Though crafting code is one particular Portion of growth, being aware of tips on how to communicate with it successfully all through execution is Similarly essential. Present day improvement environments occur Outfitted with potent debugging abilities — but several developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, step through code line by line, and even modify code about the fly. When utilized effectively, they Allow you to notice just how your code behaves throughout execution, and that is invaluable for tracking down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for entrance-conclusion builders. They let you inspect the DOM, observe network requests, watch real-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change discouraging UI issues into manageable tasks.
For backend or procedure-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command around operating procedures and memory administration. Understanding these tools might have a steeper Mastering curve but pays off when debugging general performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become relaxed with version Manage units like Git to comprehend code heritage, find the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools implies heading over and above default options and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when challenges come up, you’re not misplaced at nighttime. The higher you recognize your instruments, the greater time it is possible to expend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before jumping into the code or earning guesses, builders want to create a consistent ecosystem or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of likelihood, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater detail you might have, the simpler it results in being to isolate the exact situations under which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same information, simulating very similar consumer interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated checks that replicate the edge conditions or state transitions included. These checks not just enable expose the problem but in addition stop regressions Sooner or later.
Sometimes, the issue could possibly be environment-certain — it would materialize only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a step — it’s a attitude. It calls for patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, You should utilize your debugging applications extra effectively, test possible fixes safely and securely, and converse far more Plainly with all your team or users. It turns an abstract grievance right into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Mistake Messages
Error messages are sometimes the most precious clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, developers should master to take care of error messages as direct communications from the procedure. They generally show you just what exactly occurred, exactly where it happened, and in some cases even why it took place — if you understand how to interpret them.
Commence by looking through the message carefully As well as in total. Many builders, especially when less than time pressure, look at the initial line and immediately start out generating assumptions. But deeper from the error stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it level to a selected file and line range? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also beneficial to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can dramatically hasten your debugging procedure.
Some problems are imprecise or generic, and in Individuals conditions, it’s essential to examine the context in which the error transpired. Test related log entries, input values, and recent changes inside the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood while not having to pause execution or phase in the code line by line.
A very good logging system begins with understanding what to log and at what level. Typical logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common events (like successful get started-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and decelerate your technique. Concentrate on key occasions, point out alterations, input/output values, and important selection points as part of your code.
Format your log messages Evidently and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Ultimately, smart logging is about equilibrium and clarity. By using a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot troubles, attain deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological task—it's a sort of investigation. To effectively recognize and correct bugs, builders must method the method just like a detective fixing a secret. This attitude will help stop working elaborate issues into manageable areas and observe clues logically to uncover the foundation cause.
Begin by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect just as much appropriate data as you may devoid of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be causing this actions? Have any variations recently been made into the codebase? Has this difficulty transpired ahead of below related conditions? The objective is to slender down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results direct you nearer to the truth.
Pay out shut consideration to small facts. Bugs usually hide while in the least predicted locations—similar to a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch The problem without entirely understanding it. Short term fixes could disguise the real dilemma, check here only for it to resurface later on.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn into more practical at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the simplest approaches to increase your debugging competencies and overall improvement effectiveness. Exams not just support capture bugs early and also function a security Internet that provides you self esteem when earning changes to your codebase. A nicely-examined application is easier to debug since it permits you to pinpoint just where and when a problem occurs.
Start with unit tests, which focus on person functions or modules. These small, isolated tests can quickly expose regardless of whether a particular piece of logic is Operating as expected. When a test fails, you immediately know where by to glimpse, appreciably cutting down enough time expended debugging. Unit exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Beforehand staying mounted.
Up coming, integrate integration checks and conclude-to-conclusion exams into your workflow. These assist ensure that several areas of your application do the job collectively smoothly. They’re significantly handy for catching bugs that take place in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to Assume critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code construction and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing tests turns debugging from a annoying guessing video game into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hrs, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see the issue from a new perspective.
When you're too close to the code for too prolonged, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this particular state, your brain becomes less economical at trouble-resolving. A short walk, a coffee crack, or maybe switching to a distinct activity for 10–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue when they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during lengthier debugging classes. Sitting in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue discover a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weakness—it’s a wise system. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can educate you a thing beneficial in case you make the effort to replicate and review what went wrong.
Begin by asking your self several essential issues when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like unit testing, code evaluations, or logging? The answers usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be an outstanding practice. Hold a developer journal or keep a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see designs—recurring troubles or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly potent. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. In any case, a lot of the ideal builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — nevertheless the payoff is large. It will make you a more productive, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.